[C++] 배열과 Swap함수를 활용한 연습: 로또 번호 생성기
로또 번호 생성기
인프런 Rookiss님의 'Part1: C++ 프로그래밍 입문' 강의를 기반으로 정리한 필기입니다.
😎[C++과 언리얼로 만드는 MMORPG 게임 개발 시리즈] Part1: C++ 프로그래밍 입문 강의 들으러 가기!
1. Swap 함수 만들기
void Swap(int& a, int& b) { int temp = a; a = b; b = temp; }
원본 데이터를 수정하기 위해 & 참조 타입으로 매개변수를 받는다.
2. 정렬 함수 만들기
정렬 함수 만들기 (작은 숫자가 먼저 오도록 정렬)
- { 1 ,3, 5, 6, 15, 42 } => { 1, 3, 5, 6, 15, 42 }
void Sort(int numbers[], int count) { for (int i = 0; i < count; i++) { // i번째 값이 제일 좋은 후보라고 가정 int best = i; // 다른 후보와 비교를 통해 제일 좋은 후보를 찾아나선다 for (int j = i + 1; j < count; j++) { if (numbers[j] < numbers[best]) best = j; } // 제일 좋은 후보와 교체하는 과정 if (i != best) Swap(numbers[i], numbers[best]); } }
void Sort(int numbers[], int count)
배열을 처리하는 함수에게 "배열의 종류"와 "배열 원소의 개수"를 알려주려면, 두 개의 서로 독립된 매개변수를 정보로 넘겨주어야 한다.
- "배열의 종류" int numbers[]
- "배열 원소의 개수" int count
매개변수를 넘길 때
- "배열의 종류" 의 크기는 전체 배열의 크기 = 데이터 6개 * 4byte = 24byte
- "배열 원소의 개수" 의 크기는 배열의 원소 크기 = 4byte
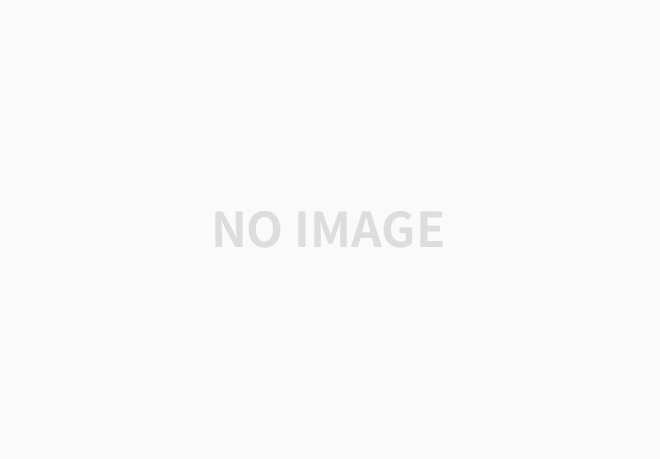
3. 로또 번호 생성
void ChooseLotto(int numbers[]) { srand((unsigned)time(0)); // 랜덤 시드 설정 int count = 0; while (count != 6) { int randValue = 1 + (rand() % 45); // 1~45 // 이미 찾은 값인지? bool found = false; for (int i = 0; i < count; i++) { // 이미 찾은 값 if (numbers[i] == randValue) { found = true; break; } } // 못 찾았으면 추가! if (found == false) { numbers[count] = randValue; count++; } } Sort(numbers, 6); }
전체 코드
더보기
#include <iostream> using namespace std; // 로또 번호 생성기 void Swap(int& a, int& b) { int temp = a; a = b; b = temp; } // 2) 정렬 함수 만들기 (작은 숫자가 먼저 오도록 정렬) // { 1 ,3, 5, 6, 15, 42 } => { 1, 3, 5, 6, 15, 42 } void Sort(int numbers[], int count) { for (int i = 0; i < count; i++) { // i번째 값이 제일 좋은 후보라고 가정 int best = i; // 다른 후보와 비교를 통해 제일 좋은 후보를 찾아나선다 for (int j = i + 1; j < count; j++) { if (numbers[j] < numbers[best]) best = j; } // 제일 좋은 후보와 교체하는 과정 if (i != best) Swap(numbers[i], numbers[best]); } } // 3) 로또 번호 생성 void ChooseLotto(int numbers[]) { srand((unsigned)time(0)); // 랜덤 시드 설정 int count = 0; while (count != 6) { int randValue = 1 + (rand() % 45); // 1~45 // 이미 찾은 값인지? bool found = false; for (int i = 0; i < count; i++) { // 이미 찾은 값 if (numbers[i] == randValue) { found = true; break; } } // 못 찾았으면 추가! if (found == false) { numbers[count] = randValue; count++; } } Sort(numbers, 6); } int main() { // 1) Swap 함수 만들기 int a = 1; int b = 2; Swap(a, b); // a = 2, b = 1 cout << a << " " << b << endl; // 2) 정렬 함수 만들기 (작은 숫자가 먼저 오도록 정렬) int numbers[6] = { 1, 42, 3, 15, 5 ,6 }; int size1 = sizeof(numbers); // 6개의 숫자 * 4바이트 = 24바이트 int size2 = sizeof(int); // int형 4바이트 Sort(numbers, 6); // sizeof(numbers) / sizeof(int) // ex) 24바이트 / 4바이트 = 6개의 숫자 // { 1 ,3, 5, 6, 15, 42 } // 3) 로또 번호 생성 ChooseLotto(numbers); for (int i = 0; i < 6; i++) cout << numbers[i] << endl; return 0; }
실행화면
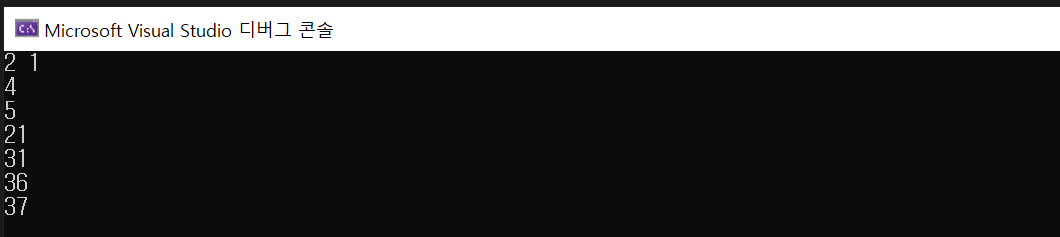
'⭐ Programming > C++' 카테고리의 다른 글
[C++] 다차원 배열, 2차원 배열 (0) | 2022.03.27 |
---|---|
[C++] 다중 포인터 (이중 포인터) (0) | 2022.03.27 |
[C++] 포인터 vs 배열 (0) | 2022.03.26 |
[C++] 배열 기초 (0) | 2022.03.26 |
[C++] 포인터 vs 참조, const (0) | 2022.03.26 |
댓글
이 글 공유하기
다른 글
-
[C++] 다차원 배열, 2차원 배열
[C++] 다차원 배열, 2차원 배열
2022.03.27 -
[C++] 다중 포인터 (이중 포인터)
[C++] 다중 포인터 (이중 포인터)
2022.03.27 -
[C++] 포인터 vs 배열
[C++] 포인터 vs 배열
2022.03.26 -
[C++] 배열 기초
[C++] 배열 기초
2022.03.26
댓글을 사용할 수 없습니다.