[UE] Console, DrawDebugLine
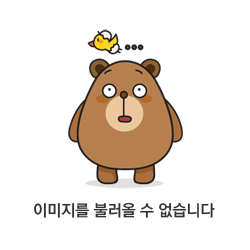
언리얼 엔진에서 콘솔은 사용자가 실시간으로 명령을 입력하고 기능을 실행할 수 있게 해주는 개발자 도구이다. 개발 중에 게임을 테스트하고 디버깅하는 방법을 제공하는 중요한 기능이다. 콘솔은 게임 내 또는 편집기에서 물결표 키(~)를 눌러 액세스할 수 있다. 콘솔이 열리면 게임 설정 변경, 액터 스폰 또는 사용자 지정 스크립트 실행과 같은 다양한 작업을 수행할 수 있는 명령을 입력할 수 있다.
목차
Plugins | ||
Example | ||
Example.Build.cs ExampleConsoleCommand.h .cpp 생성 ExampleDebuggerCategory.h .cpp ExampleModule.h .cpp |
||
Console 개념정리
언리얼 엔진의 몇 가지 일반적인 콘솔 명령:
stat fps: 현재 프레임 속도 및 기타 성능 통계를 표시한다.
collision 표시: 게임의 모든 액터에 대한 충돌 메시를 표시한다.
killall: 특정 액터 클래스의 모든 인스턴스를 파괴한다.
r.setres: 게임 창의 해상도를 설정한다.
이러한 기본 제공 명령 외에도 사용자는 사용자 지정 콘솔 명령을 만들어 게임 내에서 특정 작업을 수행할 수 있다.
전반적으로 콘솔은 언리얼 엔진으로 작업하는 개발자에게 필수적인 도구로, 전체 프로젝트를 다시 컴파일하고 다시 시작하지 않고도 게임을 빠르게 테스트하고 디버그할 수 있는 방법을 제공한다.
Alpha Blend 연산
(1, 0, 0, 0.25), (0, 0, 1, 1)
해당 두 값이 주어졌을때
Src*A | Dest * B | Src*A + Dest * B |
(1, 0, 0) * Src.a = (1, 0, 0) * 0.25 |
(0, 0, 1) * (1 -Src.a) = (0, 0, 1) * (1 - 0.25) |
(Src*A + Dest * B)은 선형보간 연산과 같다. |
(0.25, 0, 0) | (0, 0, 0.75) | (0.25, 0, 0.75) |
※ a = alpha
HAL(Hardware Abstraction Layer), RHI (Render Hardware Interface)
↑ ↑ ↑
HAL HAL HAL ...
↑ ↗ ↗
RHI (Render Hardware Interface)
↕
Draw
ConsoleManager에 HAL이 들어간다.
GameMode 상황에서의 명령창
GameMode 상황에서의 명령창
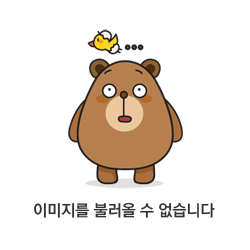
`를 눌러 명령창 실행
- DisableEQFilter
- DisableAllScreenMessages
- etc.. 다양한 명령을 수행할 수 있다.
Console
ExampleConsoleCommand 생성
새 C++ 클래스 - 없음 - ExampleConsoleCommand 생성
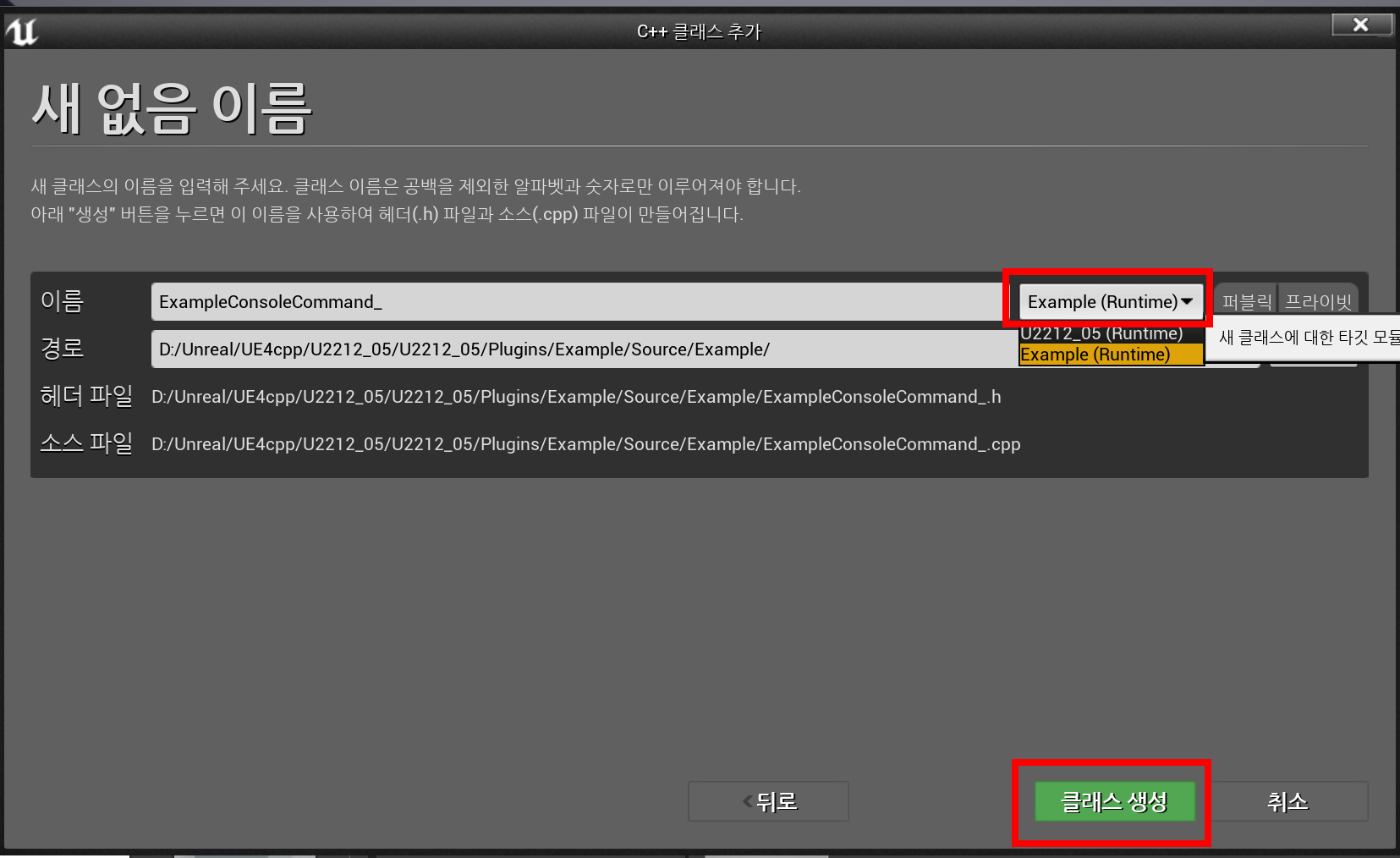
ExampleConsoleCommand.h
#pragma once #include "CoreMinimal.h" class EXAMPLE_API FExampleConsoleCommand { public: FExampleConsoleCommand(); ~FExampleConsoleCommand(); private: struct IConsoleCommand* Command; };
ExampleConsoleCommand.cpp
#include "ExampleConsoleCommand.h" #include "HAL/IConsoleManager.h" FExampleConsoleCommand::FExampleConsoleCommand() { } FExampleConsoleCommand::~FExampleConsoleCommand() { }
ExampleModule
ExampleModule.h
#pragma once #include "CoreMinimal.h" #include "Modules/ModuleManager.h" class FExampleModule : public IModuleInterface { public: virtual void StartupModule() override; virtual void ShutdownModule() override; private: TSharedPtr<class FExampleConsoleCommand> ConsoleCommand; };
변수 추가
- TSharedPtr<class FExampleConsoleCommand> ConsoleCommand;
ExampleModule.cpp
#include "ExampleModule.h" #include "ExampleDubuggerCategory.h" #include "ExampleConsoleCommand.h" //헤더 추가 #include "GameplayDebugger.h" #define LOCTEXT_NAMESPACE "FExampleModule" IMPLEMENT_MODULE(FExampleModule, Example)//모듈의 시작점을 알려준다. void FExampleModule::StartupModule() { //Debugger { IGameplayDebugger::FOnGetCategory category; category.BindStatic(&FExampleDubuggerCategory::MakeInstance); IGameplayDebugger::Get().RegisterCategory("Example", category, EGameplayDebuggerCategoryState::EnabledInGameAndSimulate, 5); //5번 슬롯 할당 IGameplayDebugger::Get().NotifyCategoriesChanged(); } //Console Command { ConsoleCommand = MakeShareable(new FExampleConsoleCommand()); } } void FExampleModule::ShutdownModule() { if (IGameplayDebugger::IsAvailable()) IGameplayDebugger::Get().UnregisterCategory("Example"); if (ConsoleCommand.IsValid()) ConsoleCommand.Reset(); //스마트포인터를 제거할 수 있도록 Reset()을 콜 해준다. 자동화가 문제가 되는경우가 있으므로 넣어준다. } #undef LOCTEXT_NAMESPACE
StartupModule()에 Debugger, Console Command 기본값 추가
Console Command
- ConsoleCommand = MakeShareable(new FExampleConsoleCommand());
MakeShareable | SharedPtr, Shared Ref 둘 다 생성 가능 |
MakeShared | 기존에 포인터가 있는 상황에서 Shared Pointer를 만들 때 사용. |
MakeSharedRef | Shared Reference 전용 |
ExampleDebuggerCategory
ExampleDebuggerCategory.h
#pragma once #include "CoreMinimal.h" #include "GameplayDebuggerCategory.h" class EXAMPLE_API FExampleDubuggerCategory : public FGameplayDebuggerCategory { public: FExampleDubuggerCategory(); ~FExampleDubuggerCategory(); public: static TSharedRef<FGameplayDebuggerCategory> MakeInstance(); public: void CollectData(APlayerController* OwnerPC, AActor* DebugActor) override; void DrawData(APlayerController* OwnerPC, FGameplayDebuggerCanvasContext& CanvasContext) override; //실제로 그려주는 역할 private: struct FCategoryData { bool bDraw = false; //그릴지 말지 FString Name; //객체 이름 FVector Location;//객체 위치 FVector Forward; //객체 전방벡터 }; private: FCategoryData PlayerPawnData; //Player 정보 FCategoryData ForwardActorData;//Plyaer 전방의 다음 객체 FCategoryData DebugActorData; //Debug 객체 private: float TraceDistance = 500; };
구조체 생성
- struct FCategoryData
변수 생성
- FCategoryData PlayerPawnData;
- FCategoryData ForwardActorData;
- FCategoryData DebugActorData;
- float TraceDistance
ExampleDebuggerCategory.cpp
#include "ExampleDubuggerCategory.h" #include "CanvasItem.h" #include "DrawDebugHelpers.h" #include "GameFramework/Character.h" #include "GameFramework/PlayerController.h" FExampleDubuggerCategory::FExampleDubuggerCategory() { bShowOnlyWithDebugActor = false; //bShowOnlyWithDebugActor는 DebugActor만 보여줘라만 의미. False로 기본값을 설정해 다 보여주도록 바꿔준다. } FExampleDubuggerCategory::~FExampleDubuggerCategory() { } TSharedRef<FGameplayDebuggerCategory> FExampleDubuggerCategory::MakeInstance() { return MakeShareable(new FExampleDubuggerCategory()); } void FExampleDubuggerCategory::CollectData(APlayerController * OwnerPC, AActor * DebugActor) { FGameplayDebuggerCategory::CollectData(OwnerPC, DebugActor); //GLog->Log(OwnerPC->GetPawn()->GetName()); //if (!!DebugActor) // GLog->Log(DebugActor->GetName()); ACharacter* player = OwnerPC->GetPawn<ACharacter>(); //Player를 가져온다. if (player == nullptr) return; //Player가 없다면 끝낸다. //Player { PlayerPawnData.bDraw = true; PlayerPawnData.Name = player->GetName(); PlayerPawnData.Location = player->GetActorLocation(); PlayerPawnData.Forward = player->GetActorForwardVector(); } //Forward Actor { FHitResult hitResult; FVector start = PlayerPawnData.Location; //시작 지점 = PlayerPawn 위치 FVector end = start + player->GetActorForwardVector() * TraceDistance;//끝 지점 = 시작 지점 + TraceDistance만큼 지점 FCollisionQueryParams params; //충돌 parameters params.AddIgnoredActor(player);//player는 충돌에서 배제한다. player->GetWorld()->LineTraceSingleByChannel(hitResult, start, end, ECollisionChannel::ECC_Visibility, params); //ECC_Visibility는 보이는것 다 추적하겠다는 의미 if(hitResult.bBlockingHit) { ForwardActorData.bDraw = true; ForwardActorData.Name = hitResult.GetActor()->GetName(); ForwardActorData.Location = hitResult.GetActor()->GetActorLocation(); ForwardActorData.Forward = hitResult.GetActor()->GetActorForwardVector(); } } //DebugActor if (!!DebugActor) { DebugActorData.bDraw = true; DebugActorData.Name = DebugActor->GetName(); DebugActorData.Location = DebugActor->GetActorLocation(); DebugActorData.Forward = DebugActor->GetActorForwardVector(); } } void FExampleDubuggerCategory::DrawData(APlayerController* OwnerPC, FGameplayDebuggerCanvasContext& CanvasContext) //여기서 CanvasContext는 Canvas 객체 { FGameplayDebuggerCategory::DrawData(OwnerPC, CanvasContext); FVector start = PlayerPawnData.Location; //시작 지점 FVector end = start + PlayerPawnData.Forward * TraceDistance; //끝 지점 DrawDebugLine(OwnerPC->GetWorld(), start, end, FColor::Red);//시작부터 끝 지점까지 빨간선으로 그려서 출력 FCanvasTileItem item(FVector2D(10, 10), FVector2D(300, 215), FLinearColor(0, 0, 0, 0.25f));//출력시작위치(좌상단), 출력사이즈, 색 item.BlendMode = ESimpleElementBlendMode::SE_BLEND_AlphaBlend;//어떻게 블랜드할지//AlphaBlend형식으로 블랜드하겠다. CanvasContext.DrawItem(item, CanvasContext.CursorX, CanvasContext.CursorY);//Canvas객체에 item을 그려준다. 이 때 마우스커서 X, Y 위치를 출력한다. CanvasContext.Printf(FColor::Green, L" -- Player Pawn --"); CanvasContext.Printf(FColor::White, L" Name : %s", *PlayerPawnData.Name); CanvasContext.Printf(FColor::White, L" Location : %s", *PlayerPawnData.Location.ToString()); CanvasContext.Printf(FColor::White, L" Forward : %s", *PlayerPawnData.Forward.ToString()); CanvasContext.Printf(FColor::White, L""); if (ForwardActorData.bDraw) //ForwardActor가 있다면 { CanvasContext.Printf(FColor::Green, L" -- Forward Actor --"); CanvasContext.Printf(FColor::White, L" Name : %s", *ForwardActorData.Name); CanvasContext.Printf(FColor::White, L" Location : %s", *ForwardActorData.Location.ToString()); CanvasContext.Printf(FColor::White, L" Forward : %s", *ForwardActorData.Forward.ToString()); CanvasContext.Printf(FColor::White, L""); } if (DebugActorData.bDraw) //DebugActor가 있다면 { CanvasContext.Printf(FColor::Green, L" -- Select Actor --"); CanvasContext.Printf(FColor::White, L" Name : %s", *DebugActorData.Name); CanvasContext.Printf(FColor::White, L" Location : %s", *DebugActorData.Location.ToString()); CanvasContext.Printf(FColor::White, L" Forward : %s", *DebugActorData.Forward.ToString()); } }
실행화면
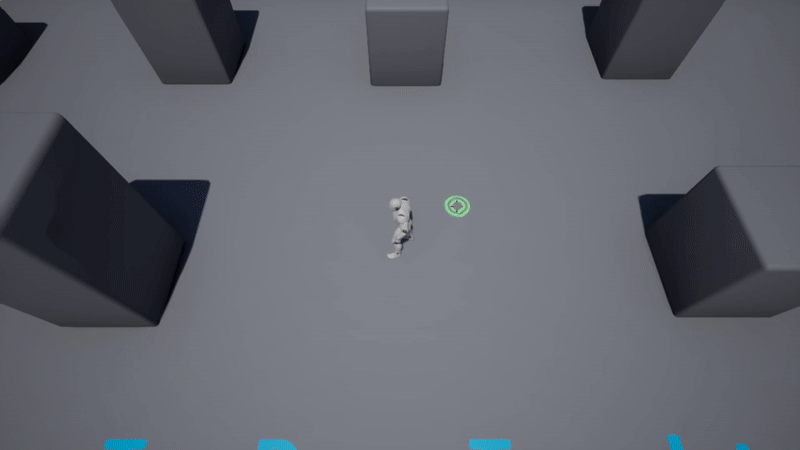
' 눌러서 확인
F8로 빠져나온 후 빙의하여 큐브 오브젝트를 누른 후에 다시 인게임으로 들어가면 Select Actor를 확인할 수 있다.
'⭐ Unreal Engine > UE Plugin - Basic' 카테고리의 다른 글
[UE] Plugin (Save StaticMesh & RenderData, LOD) (0) | 2023.04.12 |
---|---|
[UE] Plugin (StaticMesh Detail) (0) | 2023.04.07 |
[UE] StaticMesh (0) | 2023.04.06 |
[UE] Console Command (0) | 2023.04.05 |
[UE] Plugin, Slate UI (0) | 2023.04.03 |
댓글
이 글 공유하기
다른 글
-
[UE] Plugin (StaticMesh Detail)
[UE] Plugin (StaticMesh Detail)
2023.04.07플러그인 모듈 생성 플러그인 모듈은 플러그인의 기능을 구현하는 C++ 코드다. 모듈은 모든 Unreal Engine 모듈과 동일한 방식으로 작동하며, 헤더 파일과 소스 파일로 구성된다. Unreal Editor에서 "Add New -> C++ Class"를 선택하고 "Module Class"를 선택하여 모듈 클래스를 만들 수 있다. 목차 Plugins Example Example.Build.csExampleConsoleCommand.h .cpp ExampleDebuggerCategory.h .cppExampleModule.h .cppStaticMesh_Detail.h .cppSource Utilities CHelper.hCLog.h .cpp Global.hCStaticMesh.h .cpp…. -
[UE] StaticMesh
[UE] StaticMesh
2023.04.06플러그인 시스템을 통해 개발자는 Unreal Editor 내에서 StaticMesh에 적용할 수 있는 새로운 도구, 수정자 및 렌더링 옵션을 생성할 수 있으며 이러한 새로운 기능을 다른 개발자 또는 더 넓은 커뮤니티와 쉽게 공유할 수 있다. StaticMesh 플러그인을 만들려면 개발자는 먼저 Unreal의 Asset Editor를 사용하여 수행할 수 있는 새 자산 유형을 정의해야 한다. 이 새로운 자산 유형은 새로운 재료 또는 충돌 모양과 같은 사용자 정의 속성 및 기능으로 확장될 수 있다. 플러그인이 생성되면 다른 프로젝트에서 사용할 수 있도록 패키징 및 배포할 수 있으며, 개발자는 언리얼 에디터의 사용자 인터페이스를 통해 자신의 StaticMesh에 새 기능을 쉽게 추가할 수 있다. 전반적으로 Un… -
[UE] Console Command
[UE] Console Command
2023.04.05콘솔이 열리면 게임 설정 변경, 액터 스폰 또는 사용자 지정 스크립트 실행과 같은 다양한 작업을 수행할 수 있는 명령을 입력할 수 있다. 전반적으로 콘솔은 언리얼 엔진으로 작업하는 개발자에게 필수적인 도구로, 전체 프로젝트를 다시 컴파일하고 다시 시작하지 않고도 게임을 빠르게 테스트하고 디버그할 수 있는 방법을 제공한다. 목차 Plugins Example Example.Build.csExampleConsoleCommand.h .cpp ExampleDebuggerCategory.h .cppExampleModule.h .cpp Console Command FModuleManager::LoadModuleChecked FModuleManager::LoadModuleChecked("__Pr… -
[UE] Plugin, Slate UI
[UE] Plugin, Slate UI
2023.04.03언리얼 엔진에서 플러그인은 엔진의 기능을 확장하는 모듈식 구성요소이다. 사용자 정의 플러그인을 생성하여 새로운 기능을 추가하거나 기존 기능을 수정할 수 있으므로 개발자가 특정 요구 사항에 맞게 엔진을 조정할 수 있다. 커스텀 플러그인을 만들 때 모듈식 디자인의 모범 사례를 따르고 언리얼 엔진의 프로그래밍 규칙을 준수하는 것이 중요하다. 목차 Plugins Example Example.Build.csExampleModule.h .cppExampleDebuggerCategory.h .cpp 생성 Slate UI 언리얼 엔진에서 커스텀 플러그인을 만들기언리얼 에디터를 열고 "플러그인" 메뉴로 이동한다."새 플러그인"을 클릭하고 생성할 플러그인 유형을 선택한다.플러그인의 이름과 위치를 선택하…
댓글을 사용할 수 없습니다.