[DirectX11] 010 World
목차
World
World에 3개의 사각형 출력 후 크기 조절하기 및 위치 이동하기
World.fx 코드
10_World.fx
더보기
matrix World; matrix View; matrix Projection; uint Index; struct VertexInput { float4 Position : Position; }; struct VertexOutput { float4 Position : SV_Position; }; VertexOutput VS(VertexInput input) { VertexOutput output; output.Position = mul(input.Position, World); output.Position = mul(output.Position, View); output.Position = mul(output.Position, Projection); return output; } float4 PS_R(VertexOutput input) : SV_Target { if (Index == 0) return float4(1, 0, 0, 1); if (Index == 1) return float4(0, 1, 0, 1); if (Index == 2) return float4(0, 0, 1, 1); return float4(0, 0, 0, 1); } RasterizerState FillMode_Wireframe { FillMode = Wireframe; }; technique11 T0 { pass P0 { SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS_R())); } pass P1 { SetRasterizerState(FillMode_Wireframe); SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS_R())); } }
uint Index;
// 사각형 인덱스별로 색깔 다르게 설정
float4 PS_R(VertexOutput input) : SV_Target
{
if (Index == 0)
return float4(1, 0, 0, 1);
if (Index == 1)
return float4(0, 1, 0, 1);
if (Index == 2)
return float4(0, 0, 1, 1);
return float4(0, 0, 0, 1);
}
WorldDemo2 코드
WorldDemo2.h
더보기
#pragma once #include "Systems/IExecute.h" class WorldDemo2 : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtual void PostRender() override {} virtual void ResizeScreen() override {} private: Shader* shader; Vertex vertices[6]; ID3D11Buffer* vertexBuffer; UINT index = 0; Matrix world[3]; };
WorldDemo2.cpp
더보기
#include "stdafx.h" #include "WorldDemo2.h" void WorldDemo2::Initialize() { shader = new Shader(L"10_World.fx"); //Local vertices[0].Position = Vector3(+0.0f, +0.0f, 0.0f); vertices[1].Position = Vector3(+0.0f, +0.5f, 0.0f); vertices[2].Position = Vector3(+0.5f, +0.0f, 0.0f); vertices[3].Position = Vector3(+0.5f, +0.0f, 0.0f); vertices[4].Position = Vector3(+0.0f, +0.5f, 0.0f); vertices[5].Position = Vector3(+0.5f, +0.5f, 0.0f); D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(Vertex) * 6; desc.BindFlags = D3D11_BIND_VERTEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = vertices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &vertexBuffer)); for (int i = 0; i < 3; i++) D3DXMatrixIdentity(&world[i]); } void WorldDemo2::Destroy() { SafeDelete(shader); SafeRelease(vertexBuffer); } void WorldDemo2::Update() { ImGui::InputInt("Select", (int *)&index); index %= 3; if (Keyboard::Get()->Press(VK_CONTROL)) { if (Keyboard::Get()->Press(VK_RIGHT)) { world[index]._41 += 2.0f * Time::Delta(); } else if (Keyboard::Get()->Press(VK_LEFT)) { world[index]._41 -= 2.0f * Time::Delta(); } } else { if (Keyboard::Get()->Press(VK_RIGHT)) { world[index]._22 += 2.0f * Time::Delta(); } else if (Keyboard::Get()->Press(VK_LEFT)) { world[index]._22 -= 2.0f * Time::Delta(); } } } void WorldDemo2::Render() { shader->AsMatrix("View")->SetMatrix(Context::Get()->View()); shader->AsMatrix("Projection")->SetMatrix(Context::Get()->Projection()); UINT stride = sizeof(Vertex); UINT offset = 0; D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset); D3D::GetDC()->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST); shader->AsScalar("Index")->SetInt(0); shader->AsMatrix("World")->SetMatrix(world[0]); shader->Draw(0, 0, 6); shader->AsScalar("Index")->SetInt(1); shader->AsMatrix("World")->SetMatrix(world[1]); shader->Draw(0, 0, 6); shader->AsScalar("Index")->SetInt(2); shader->AsMatrix("World")->SetMatrix(world[2]); shader->Draw(0, 0, 6); }
Main.cpp
더보기
#include "stdafx.h" #include "Main.h" #include "Systems/Window.h" #include "WorldDemo2.h" #include "WorldDemo.h" #include "UserInterfaceDemo.h" #include "RectDemo.h" #include "TriangleList.h" #include "Vertex_Line2.h" #include "Vertex_Line.h" void Main::Initialize() { Push(new WorldDemo2()); } void Main::Ready() { } void Main::Destroy() { for (IExecute* exe : executes) { exe->Destroy(); SafeDelete(exe); } } void Main::Update() { for (IExecute* exe : executes) exe->Update(); } void Main::PreRender() { for (IExecute* exe : executes) exe->PreRender(); } void Main::Render() { for (IExecute* exe : executes) exe->Render(); } void Main::PostRender() { for (IExecute* exe : executes) exe->PostRender(); } void Main::ResizeScreen() { for (IExecute* exe : executes) exe->ResizeScreen(); } void Main::Push(IExecute * execute) { executes.push_back(execute); execute->Initialize(); } int WINAPI WinMain(HINSTANCE instance, HINSTANCE prevInstance, LPSTR param, int command) { D3DDesc desc; desc.AppName = L"D3D Game"; desc.Instance = instance; desc.bFullScreen = false; desc.bVsync = false; desc.Handle = NULL; desc.Width = 1280; desc.Height = 720; desc.Background = Color(0.3f, 0.3f, 0.3f, 1.0f); D3D::SetDesc(desc); Main* main = new Main(); WPARAM wParam = Window::Run(main); SafeDelete(main); return wParam; }
실행화면
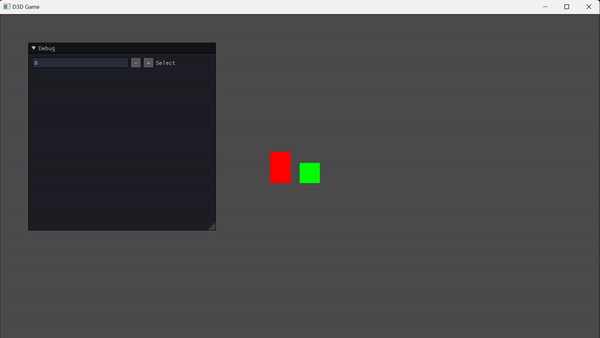
'⭐ DirectX > DirectX11 3D' 카테고리의 다른 글
[DirectX11] 012 Grid (1) | 2023.01.10 |
---|---|
[DirectX11] 011 Index (0) | 2023.01.10 |
[DirectX11] 009 World Matrix (0) | 2023.01.08 |
[DirectX11] 008 User Interface (1) | 2023.01.07 |
[DirectX11] 006~007 사각형 띄우기 (0) | 2023.01.06 |
댓글
이 글 공유하기
다른 글
-
[DirectX11] 012 Grid
[DirectX11] 012 Grid
2023.01.10목차 Grid Grid Vertex Count = ( width + 1 ) * ( height + 1 ) Index Count = ( width) * ( height ) * 6 GridDemo 코드 10_World.fx 코드는 이전글과 동일 GridDemo.h 더보기 #pragma once #include "Systems/IExecute.h" class GridDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() ove… -
[DirectX11] 011 Index
[DirectX11] 011 Index
2023.01.10목차 Index Index를 활용한 사각형 그리기 0 1 2 2 1 3 순서로 그린다. IndexDemo 코드 10_World.fx 코드는 이전글과 동일 IndexDemo.h 더보기 #pragma once #include "Systems/IExecute.h" class IndexDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtua… -
[DirectX11] 009 World Matrix
[DirectX11] 009 World Matrix
2023.01.08목차 World Matrix View Projection 행렬 4x4 행렬Identity1 0 0 00 1 0 00 0 1 00 0 0 1 World.fx 코드 08_World.fx더보기matrix World;matrix View;matrix Projection;struct VertexInput{ float4 Position : Position;};struct VertexOutput{ float4 Position : SV_Position;};float4 VS(VertexInput input) : SV_Position{ // float4의 위치(=input.Position)에 W V P를 곱하여 output.Position 반환 VertexOutput outpu… -
[DirectX11] 008 User Interface
[DirectX11] 008 User Interface
2023.01.07목차 User Interface 파이프라인 Vertex -> IA -> VS -> RS -> PS -> Pixel IA (Input Assembler)스테이지를 이용해 렌더링 데이터 세팅VS(Vertex Shader)W, V, P. 정점의 위치 변환. NDC 공간의 사용.RS(Rasterization Shader)정점을 픽셀로 바꿔준다. 픽셀로 채워주는 과정PS(Pixel Shader)한점 한점을 어떻게 처리할 것인가? 픽셀 하나하나를 처리하는 과정. 색을 결정하는 과정에 쓰인다. User Interface UserInterfaceDemo.h더보기#pragma once#include "Systems/IExecute.h"class UserInterfaceDemo : public IExe…
댓글을 사용할 수 없습니다.