[DirectX11] 012 Grid
목차
Grid
Grid
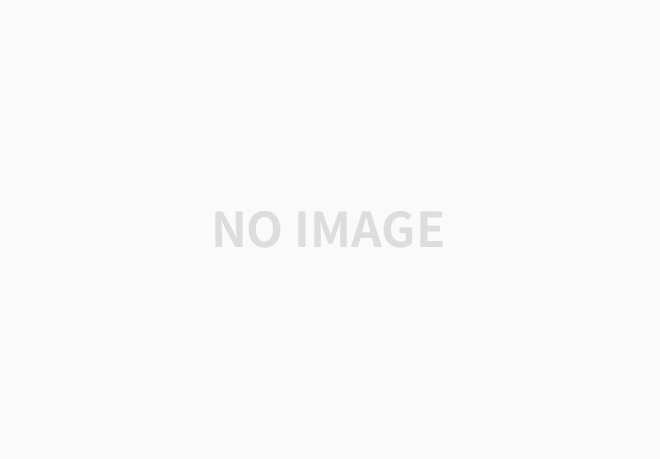
Vertex Count = ( width + 1 ) * ( height + 1 )
Index Count = ( width) * ( height ) * 6
GridDemo 코드
10_World.fx 코드는 이전글과 동일
GridDemo.h
더보기
#pragma once #include "Systems/IExecute.h" class GridDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtual void PostRender() override {} virtual void ResizeScreen() override {} private: Shader* shader; UINT width = 3, height = 3; UINT vertexCount; Vertex* vertices; ID3D11Buffer* vertexBuffer; UINT indexCount; UINT* indices; ID3D11Buffer* indexBuffer; Matrix world; };
width, height 값 변경으로 사각형 그리드 크기변경 가능
GridDemo.cpp
더보기
#include "stdafx.h" #include "GridDemo.h" void GridDemo::Initialize() { shader = new Shader(L"10_World.fx"); vertexCount = (width + 1) * (height + 1); vertices = new Vertex[vertexCount]; for (UINT y = 0; y <= height; y++) { for (UINT x = 0; x <= width; x++) { UINT i = (width + 1) * y + x; vertices[i].Position.x = (float)x; vertices[i].Position.y = (float)y; vertices[i].Position.z = 0.0f; } } //Create Vertex Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(Vertex) * vertexCount; desc.BindFlags = D3D11_BIND_VERTEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = vertices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &vertexBuffer)); } indexCount = (width * height) * 6; indices = new UINT[indexCount]; UINT index = 0; for (UINT y = 0; y < height; y++) { for (UINT x = 0; x < width; x++) { indices[index + 0] = (width + 1) * y + x; indices[index + 1] = (width + 1) * (y + 1) + x; indices[index + 2] = (width + 1) * y + x + 1; indices[index + 3] = (width + 1) * y + x + 1; indices[index + 4] = (width + 1) * (y + 1) + x; indices[index + 5] = (width + 1) * (y + 1) + x + 1; index += 6; } } //Create Index Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(UINT) * indexCount; desc.BindFlags = D3D11_BIND_INDEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = indices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &indexBuffer)); } SafeDeleteArray(vertices); SafeDeleteArray(indices); D3DXMatrixIdentity(&world); } void GridDemo::Destroy() { SafeDelete(shader); SafeDelete(vertexBuffer); SafeDelete(indexBuffer); } void GridDemo::Update() { } void GridDemo::Render() { shader->AsScalar("Index")->SetInt(0); shader->AsMatrix("World")->SetMatrix(world); shader->AsMatrix("View")->SetMatrix(Context::Get()->View()); shader->AsMatrix("Projection")->SetMatrix(Context::Get()->Projection()); UINT stride = sizeof(Vertex); UINT offset = 0; D3D::GetDC()->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST); D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset); D3D::GetDC()->IASetIndexBuffer(indexBuffer, DXGI_FORMAT_R32_UINT, 0); shader->DrawIndexed(0, 1, indexCount); }
Main.cpp
더보기
#include "stdafx.h" #include "Main.h" #include "Systems/Window.h" #include "GridDemo.h" void Main::Initialize() { Push(new GridDemo()); } void Main::Ready() { } void Main::Destroy() { for (IExecute* exe : executes) { exe->Destroy(); SafeDelete(exe); } } void Main::Update() { for (IExecute* exe : executes) exe->Update(); } void Main::PreRender() { for (IExecute* exe : executes) exe->PreRender(); } void Main::Render() { for (IExecute* exe : executes) exe->Render(); } void Main::PostRender() { for (IExecute* exe : executes) exe->PostRender(); } void Main::ResizeScreen() { for (IExecute* exe : executes) exe->ResizeScreen(); } void Main::Push(IExecute * execute) { executes.push_back(execute); execute->Initialize(); } int WINAPI WinMain(HINSTANCE instance, HINSTANCE prevInstance, LPSTR param, int command) { D3DDesc desc; desc.AppName = L"D3D Game"; desc.Instance = instance; desc.bFullScreen = false; desc.bVsync = false; desc.Handle = NULL; desc.Width = 1280; desc.Height = 720; desc.Background = Color(0.3f, 0.3f, 0.3f, 1.0f); D3D::SetDesc(desc); Main* main = new Main(); WPARAM wParam = Window::Run(main); SafeDelete(main); return wParam; }
실행화면
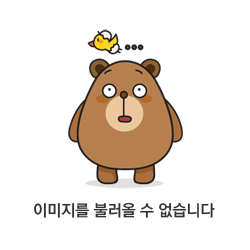
'⭐ DirectX > DirectX11 3D' 카테고리의 다른 글
[DirectX11] 015 Cube (0) | 2023.01.13 |
---|---|
[DirectX11] 013~014 Camera (1) | 2023.01.11 |
[DirectX11] 011 Index (0) | 2023.01.10 |
[DirectX11] 010 World (1) | 2023.01.09 |
[DirectX11] 009 World Matrix (0) | 2023.01.08 |
댓글
이 글 공유하기
다른 글
-
[DirectX11] 015 Cube
[DirectX11] 015 Cube
2023.01.13 -
[DirectX11] 013~014 Camera
[DirectX11] 013~014 Camera
2023.01.11 -
[DirectX11] 011 Index
[DirectX11] 011 Index
2023.01.10 -
[DirectX11] 010 World
[DirectX11] 010 World
2023.01.09
댓글을 사용할 수 없습니다.