[DirectX11] 019 Heightmap
Height Map
Terrain.fx
Terrain.fx
더보기
matrix World; matrix View; matrix Projection; Texture2D Map; struct VertexInput { float4 Position : Position; float2 Uv : Uv; }; struct VertexOutput { float4 Position : SV_Position; float2 Uv : Uv; }; VertexOutput VS(VertexInput input) { VertexOutput output; output.Position = mul(input.Position, World); output.Position = mul(output.Position, View); output.Position = mul(output.Position, Projection); output.Uv = input.Uv; return output; } SamplerState Samp; float4 PS(VertexOutput input) : SV_Target { return float4(1, 1, 1, 1); } RasterizerState FillMode_Wireframe { FillMode = Wireframe; }; technique11 T0 { pass P0 { SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS())); } pass P1 { SetRasterizerState(FillMode_Wireframe); SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS())); } }
Terrain
Terrain.h
더보기
#pragma once class Terrain { public: typedef Vertex TerrainVertex; public: Terrain(Shader* shader, wstring heightFile); ~Terrain(); void Update(); void Render(); void Pass(UINT val) { pass = val; } private: void CreateVertexData(); void CreateIndexData(); void CreateBuffer(); private: UINT pass = 0; Shader* shader; Texture* heightMap; UINT width, height; UINT vertexCount; TerrainVertex* vertices; ID3D11Buffer* vertexBuffer; UINT indexCount; UINT* indices; ID3D11Buffer* indexBuffer; };
Terrain.cpp
더보기
#include "Framework.h" #include "Terrain.h" Terrain::Terrain(Shader * shader, wstring heightFile) : shader(shader) { heightMap = new Texture(heightFile); CreateVertexData(); CreateIndexData(); CreateBuffer(); } Terrain::~Terrain() { SafeDelete(heightMap); SafeDelete(vertices); SafeDelete(indices); SafeRelease(vertexBuffer); SafeRelease(indexBuffer); } void Terrain::Update() { Matrix world; D3DXMatrixIdentity(&world); shader->AsMatrix("World")->SetMatrix(world); shader->AsMatrix("View")->SetMatrix(Context::Get()->View()); shader->AsMatrix("Projection")->SetMatrix(Context::Get()->Projection()); } void Terrain::Render() { UINT stride = sizeof(Vertex); UINT offset = 0; D3D::GetDC()->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST); D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset); D3D::GetDC()->IASetIndexBuffer(indexBuffer, DXGI_FORMAT_R32_UINT, 0); shader->DrawIndexed(0, pass, indexCount); } void Terrain::CreateVertexData() { vector<Color> heights; heightMap->ReadPixel(DXGI_FORMAT_R8G8B8A8_UNORM, &heights); width = heightMap->GetWidth(); height = heightMap->GetHeight(); vertexCount = width * height; vertices = new TerrainVertex[vertexCount]; for (UINT z = 0; z < height; z++) { for (UINT x = 0; x < height; x++) { UINT index = width * z + x; UINT pixel = width * (height - 1 - z) + x; vertices[index].Position.x = (float)x; vertices[index].Position.y = heights[index].r * 255.0f / 10.0f; vertices[index].Position.z = (float)z; } } } void Terrain::CreateIndexData() { indexCount = (width - 1) * (height - 1) * 6; indices = new UINT[indexCount]; UINT index = 0; for (UINT y = 0; y < height - 1; y++) { for (UINT x = 0; x < width - 1; x++) { indices[index + 0] = width * y + x; indices[index + 1] = width * (y + 1) + x; indices[index + 2] = width * y + x + 1; indices[index + 3] = width * y + x + 1; indices[index + 4] = width * (y + 1) + x; indices[index + 5] = width * (y + 1) + x + 1; index += 6; } } } void Terrain::CreateBuffer() { //Create Vertex Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(Vertex) * vertexCount; desc.BindFlags = D3D11_BIND_VERTEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = vertices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &vertexBuffer)); } //Create Index Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(UINT) * indexCount; desc.BindFlags = D3D11_BIND_INDEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = indices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &indexBuffer)); } }
HeightMap
HeightMapDemo.h
더보기
#pragma once #include "Systems/IExecute.h" class HeightMapDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtual void PostRender() override {} virtual void ResizeScreen() override {} private: Shader* shader; Terrain* terrain; };
HeightMapDemo.cpp
더보기
#include "stdafx.h" #include "HeightMapDemo.h" void HeightMapDemo::Initialize() { Context::Get()->GetCamera()->RotationDegree(12, 0, 0); Context::Get()->GetCamera()->Position(35, 10, -55); shader = new Shader(L"19_Terrain.fx"); terrain = new Terrain(shader, L"Terrain/Gray256.png"); terrain->Pass(1); } void HeightMapDemo::Destroy() { SafeDelete(shader); } void HeightMapDemo::Update() { terrain->Update(); } void HeightMapDemo::Render() { terrain->Render(); }
실행화면
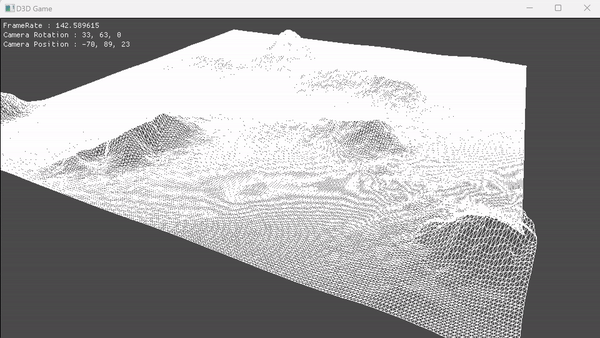
'⭐ DirectX > DirectX11 3D' 카테고리의 다른 글
[DirectX11] 023 Get Height (0) | 2023.01.20 |
---|---|
[DirectX11] 020~22 Normal Vector (0) | 2023.01.18 |
[DirectX11] 018 Texture Sampler (0) | 2023.01.16 |
[DirectX11] 017 Texture Load (0) | 2023.01.14 |
[DirectX11] 016 Texture (0) | 2023.01.14 |
댓글
이 글 공유하기
다른 글
-
[DirectX11] 023 Get Height
[DirectX11] 023 Get Height
2023.01.20 -
[DirectX11] 020~22 Normal Vector
[DirectX11] 020~22 Normal Vector
2023.01.18 -
[DirectX11] 018 Texture Sampler
[DirectX11] 018 Texture Sampler
2023.01.16 -
[DirectX11] 017 Texture Load
[DirectX11] 017 Texture Load
2023.01.14
댓글을 사용할 수 없습니다.