[DirectX11] 016 Texture
Texture
Rotation 원리
https://learn.microsoft.com/ko-kr/windows/win32/direct2d/how-to-rotate
개체를 회전하는 방법 - Win32 apps
개체를 회전하는 방법을 보여줍니다.
learn.microsoft.com
http://www.directxtutorial.com/Lesson.aspx?lessonid=9-4-5
DirectXTutorial.com
In the last lesson you built a simple, flat triangle lit with simple diffuse lighting. This triangle was not 3D, it was flat. If you managed to change it, you found it was a 2D triangle drawn in screen coordinates. The triangle you made was pre-transformed
www.directxtutorial.com
Rotation Demo
RotationDemo.h
#pragma once #include "Systems/IExecute.h" class RotationDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtual void PostRender() override {} virtual void ResizeScreen() override {} private: Shader* shader; Vertex vertices[6]; ID3D11Buffer* vertexBuffer; float z = 0.0f; Matrix world; };
RotationDemo.cpp
#include "stdafx.h" #include "RotationDemo.h" void RotationDemo::Initialize() { Context::Get()->GetCamera()->Position(0, 0, -5.0f); shader = new Shader(L"09_World.fx"); //좌측하단 기준으로 회전 //vertices[0].Position = Vector3(+0.0f, +0.0f, 0.0f); //vertices[1].Position = Vector3(+0.0f, +0.5f, 0.0f); //vertices[2].Position = Vector3(+0.5f, +0.0f, 0.0f); //vertices[3].Position = Vector3(+0.5f, +0.0f, 0.0f); //vertices[4].Position = Vector3(+0.0f, +0.5f, 0.0f); //vertices[5].Position = Vector3(+0.5f, +0.5f, 0.0f); //중앙 기준으로 회전 vertices[0].Position = Vector3(-0.5f, -0.5f, 0.0f); vertices[1].Position = Vector3(-0.5f, +0.5f, 0.0f); vertices[2].Position = Vector3(+0.5f, -0.5f, 0.0f); vertices[3].Position = Vector3(+0.5f, -0.5f, 0.0f); vertices[4].Position = Vector3(-0.5f, +0.5f, 0.0f); vertices[5].Position = Vector3(+0.5f, +0.5f, 0.0f); D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(Vertex) * 6; desc.BindFlags = D3D11_BIND_VERTEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = vertices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &vertexBuffer)); D3DXMatrixIdentity(&world); } void RotationDemo::Destroy() { SafeDelete(shader); SafeRelease(vertexBuffer); } void RotationDemo::Update() { if (Keyboard::Get()->Press(VK_RIGHT)) { z += 20 * Time::Delta(); } else if (Keyboard::Get()->Press(VK_LEFT)) { z -= 20 * Time::Delta(); } D3DXMatrixRotationZ(&world, z * Math::ToRadian(z)); } void RotationDemo::Render() { shader->AsMatrix("World")->SetMatrix(world); shader->AsMatrix("View")->SetMatrix(Context::Get()->View()); shader->AsMatrix("Projection")->SetMatrix(Context::Get()->Projection()); UINT stride = sizeof(Vertex); UINT offset = 0; D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset); D3D::GetDC()->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST); shader->Draw(0, 0, 6); }
Rotation 실행화면
좌측하단 기준 회전
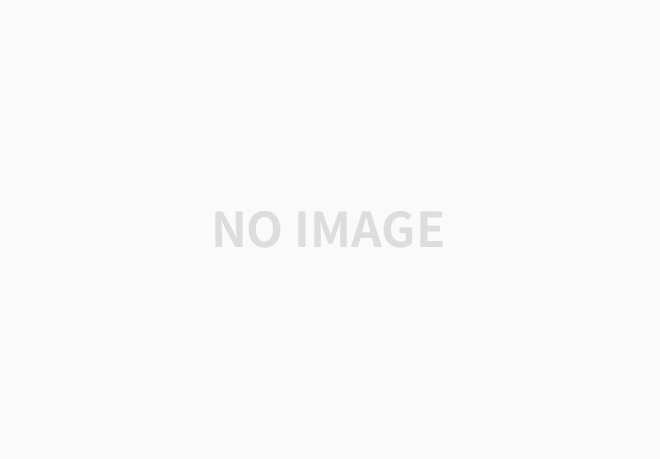
중앙 기준 회전
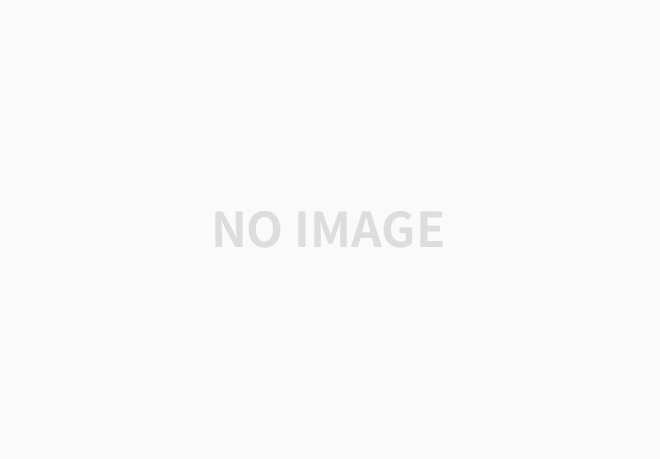
UV 좌표계
https://m.blog.naver.com/PostView.naver?isHttpsRedirect=true&blogId=sipack7297&logNo=220424401873
[D3D] 5장 : 텍스처(Texture)
텍스처(Texture) - 일반적으로 2차원 텍스처를 사용한다. 텍스처 좌표계(Texture Coordinate Sys...
blog.naver.com
Texture.fx
Texture.fx
matrix World; matrix View; matrix Projection; Texture2D Map; struct VertexInput { float4 Position : Position; float2 Uv : Uv; }; struct VertexOutput { float4 Position : SV_Position; float2 Uv : Uv; }; VertexOutput VS(VertexInput input) { VertexOutput output; output.Position = mul(input.Position, World); output.Position = mul(output.Position, View); output.Position = mul(output.Position, Projection); output.Uv = input.Uv; return output; } SamplerState Samp; float4 PS(VertexOutput input) : SV_Target { return Map.Sample(Samp, input.Uv); } RasterizerState FillMode_Wireframe { FillMode = Wireframe; }; technique11 T0 { pass P0 { SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS())); } pass P1 { SetRasterizerState(FillMode_Wireframe); SetVertexShader(CompileShader(vs_5_0, VS())); SetPixelShader(CompileShader(ps_5_0, PS())); } }
Texture2D Map; 추가
float2 Uv : Uv 추가
output.Uv = input.Uv
SamplerState Samp;
float4 PS(VertexOutput input) : SV_Target
{
return Map.Sample(Samp, input.Uv);
}
Texture
Texture.h
#pragma once #include "Systems/IExecute.h" class TextureDemo : public IExecute { public: virtual void Initialize() override; virtual void Ready() override {} virtual void Destroy() override; virtual void Update() override; virtual void PreRender() override {} virtual void Render() override; virtual void PostRender() override {} virtual void ResizeScreen() override {} private: Shader* shader; VertexTexture vertices[6]; ID3D11Buffer* vertexBuffer; UINT indices[6] = { 0, 1, 2, 2, 1, 3 }; ID3D11Buffer* indexBuffer; Matrix world; ID3D11ShaderResourceView* srv; };
VertexTexture vertices[6];
UINT indices[6] = { 0, 1, 2, 2, 1, 3};
ID3D11ShaderResourceView* srv;
Texture.cpp
#include "stdafx.h" #include "TextureDemo.h" void TextureDemo::Initialize() { Context::Get()->GetCamera()->Position(0, 0, -5.0f); shader = new Shader(L"16_Texture.fx"); vertices[0].Position = Vector3(-0.5f, -0.5f, 0.0f); vertices[1].Position = Vector3(-0.5f, +0.5f, 0.0f); vertices[2].Position = Vector3(+0.5f, -0.5f, 0.0f); vertices[3].Position = Vector3(+0.5f, +0.5f, 0.0f); vertices[0].Uv = Vector2(0, 1); vertices[1].Uv = Vector2(0, 0); vertices[2].Uv = Vector2(1, 1); vertices[3].Uv = Vector2(1, 0); //Create Vertex Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(VertexTexture) * 4; desc.BindFlags = D3D11_BIND_VERTEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = vertices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &vertexBuffer)); } //Create Index Buffer { D3D11_BUFFER_DESC desc; ZeroMemory(&desc, sizeof(D3D11_BUFFER_DESC)); desc.ByteWidth = sizeof(UINT) * 6; desc.BindFlags = D3D11_BIND_INDEX_BUFFER; D3D11_SUBRESOURCE_DATA subResource = { 0 }; subResource.pSysMem = indices; Check(D3D::GetDevice()->CreateBuffer(&desc, &subResource, &indexBuffer)); } D3DXMatrixIdentity(&world); Check(D3DX11CreateShaderResourceViewFromFile ( D3D::GetDevice(), L"../../_Textures/Box.png", NULL, NULL, &srv, NULL )); } void TextureDemo::Destroy() { SafeDelete(shader); SafeRelease(vertexBuffer); } void TextureDemo::Update() { } void TextureDemo::Render() { shader->AsMatrix("World")->SetMatrix(world); shader->AsMatrix("View")->SetMatrix(Context::Get()->View()); shader->AsMatrix("Projection")->SetMatrix(Context::Get()->Projection()); shader->AsSRV("Map")->SetResource(srv); UINT stride = sizeof(VertexTexture); UINT offset = 0; D3D::GetDC()->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset); D3D::GetDC()->IASetIndexBuffer(indexBuffer, DXGI_FORMAT_R32_UINT, 0); D3D::GetDC()->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST); shader->DrawIndexed(0, 0, 6); }
Texture 실행화면
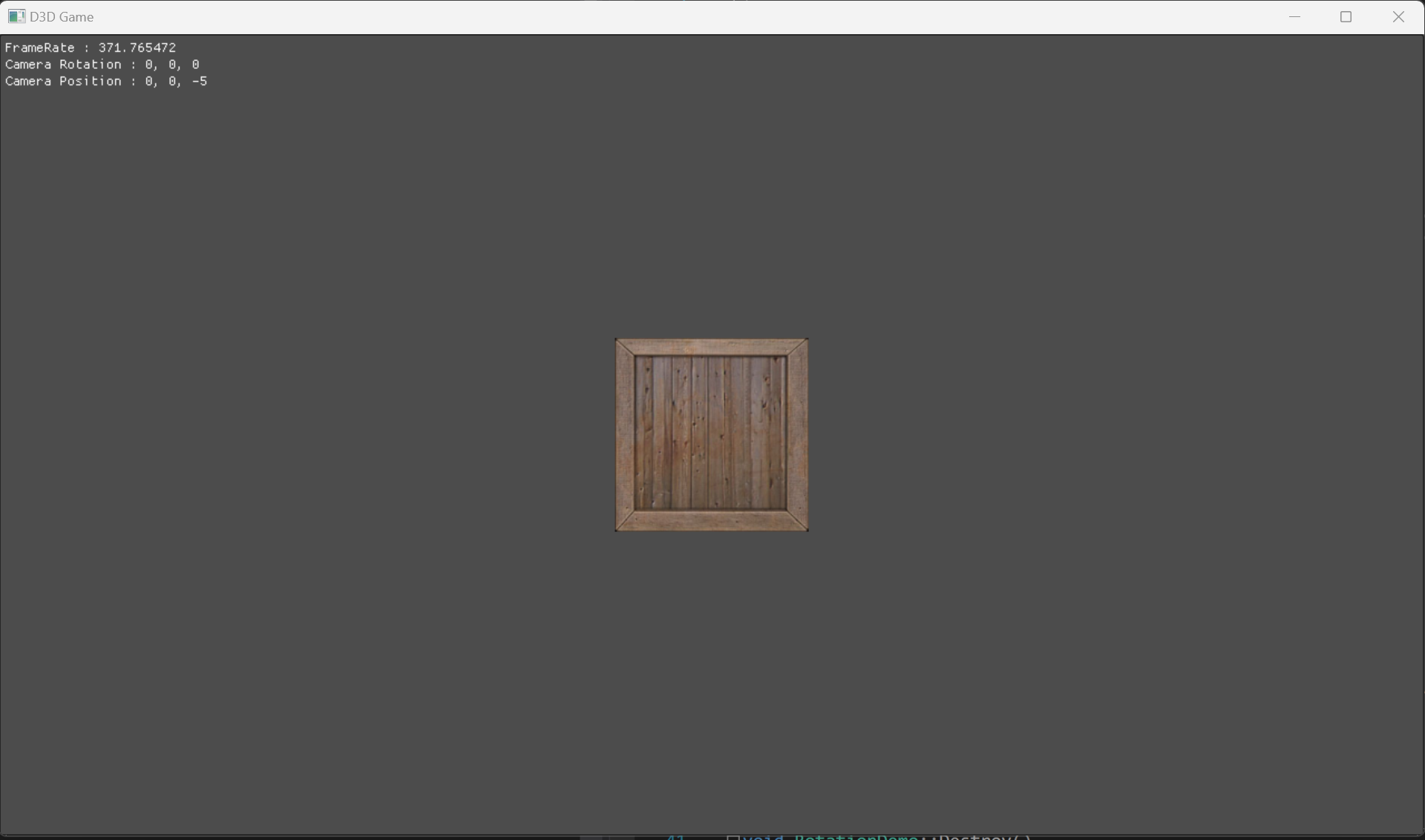
'⭐ DirectX > DirectX11 3D' 카테고리의 다른 글
[DirectX11] 018 Texture Sampler (0) | 2023.01.16 |
---|---|
[DirectX11] 017 Texture Load (0) | 2023.01.14 |
[DirectX11] 015 Cube (0) | 2023.01.13 |
[DirectX11] 013~014 Camera (0) | 2023.01.11 |
[DirectX11] 012 Grid (1) | 2023.01.10 |
댓글
이 글 공유하기
다른 글
-
[DirectX11] 018 Texture Sampler
[DirectX11] 018 Texture Sampler
2023.01.16 -
[DirectX11] 017 Texture Load
[DirectX11] 017 Texture Load
2023.01.14 -
[DirectX11] 015 Cube
[DirectX11] 015 Cube
2023.01.13 -
[DirectX11] 013~014 Camera
[DirectX11] 013~014 Camera
2023.01.11
댓글을 사용할 수 없습니다.