[UE] Interface, AnimNotify, Backstep 구현하기
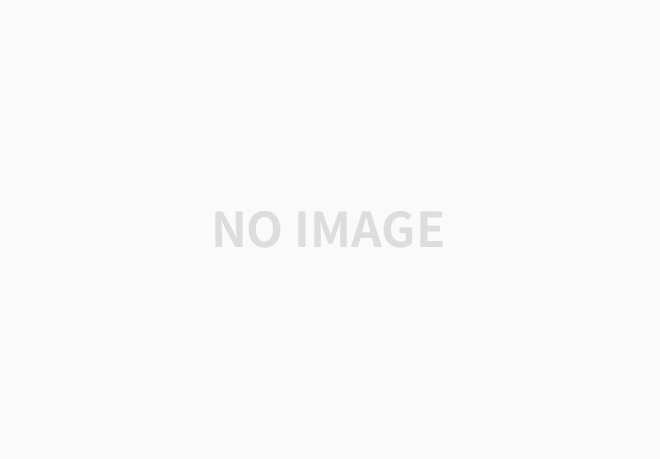
목차
Source | ||
Characters | ||
CAnimInstance.h .cpp CPlayer.h .cpp ICharacter.h .cpp 생성 |
||
Components | ||
CMontagesComponent.h .cpp CMovementComponent.h .cpp CStateComponent.h .cpp CWeaponComponent.h .cpp |
||
Notifies | ||
CAnimNotify_EndState.h .cpp 생성 | ||
Utilities | ||
CHelper.h CLog.h .cpp |
||
Weapons | ||
CAttachment.h .cpp 생성 CWeaponAsset.h .cpp 생성 |
||
Global.h CGameMode.h .cpp U2212_06.Build.cs |
||
U2212_06.uproject | ||
인터페이스
인터페이스(Interface) vs 추상클래스
인터페이스
- 인터페이스는 정의 상 순수 가상함수만 포함한다.
- C++과 Unreal C++에서 가상, 추상, 일반 모두 포함할 수 있다.
- UInterface 지정자 사용
- 그룹을 묶어주기 위해서 사용한다.
추상클래스
- 순수 가상함수를 하나라도 포함한다.
- C++과 Unreal C++에서 가상, 추상, 일반 모두 포함할 수 있다.?
- 대부분에 부모에 구현되어 있다?
- UCLASS 지정자 사용
블로그를 예시로 들면 상하위 카테고리 관계는 상속을 이용한 추상클래스, tag는 인터페이스다.
일반클래스 vs. 추상클래스
- 일반클래스는 객체화 될 수 있지만 추상클래스는 객체화 될 수 없다.
- 객체화되면 안되는 것들이 추상이다(=추상화 해야되는 대상이다).
구조체 vs. 클래스
- 접근 지정자의 차이
- 선언 시 struct, class 차이
CAnimNotify_EndState 생성
새 c++ 클래스 - AnimNotify - CAnimNotify_EndState 생성
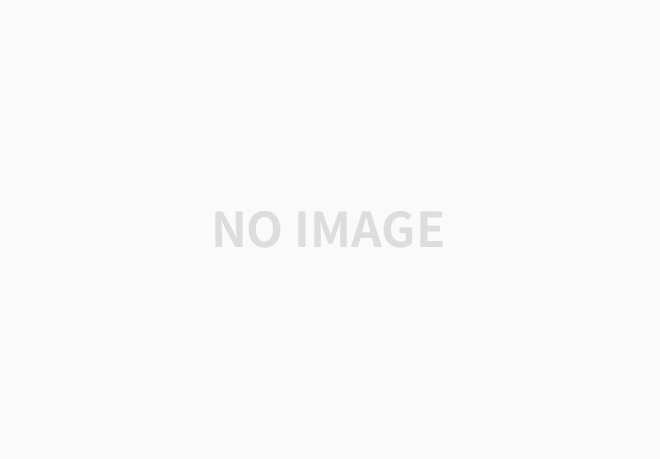
CAnimNotify_EndState.h
#pragma once #include "CoreMinimal.h" #include "Animation/AnimNotifies/AnimNotify.h" #include "Components/CStateComponent.h" #include "CAnimNotify_EndState.generated.h" UCLASS() class U2212_06_API UCAnimNotify_EndState : public UAnimNotify { GENERATED_BODY() private: UPROPERTY(EditAnywhere, Category = "Type") EStateType StateType; public: FString GetNotifyName_Implementation() const override; void Notify(USkeletalMeshComponent* MeshComp, UAnimSequenceBase* Animation) override; };
CAnimNotify_EndState.cpp
#include "CAnimNotify_EndState.h" #include "Global.h" #include "Characters/ICharacter.h" FString UCAnimNotify_EndState::GetNotifyName_Implementation() const { return "EndState"; } void UCAnimNotify_EndState::Notify(USkeletalMeshComponent* MeshComp, UAnimSequenceBase* Animation) { Super::Notify(MeshComp, Animation); CheckNull(MeshComp); CheckNull(MeshComp->GetOwner()); IICharacter* character = Cast<IICharacter>(MeshComp->GetOwner()); CheckNull(character); switch(StateType) { case EStateType::BackStep: character->End_BackStep(); break; } }
※참고) AnimNotify.h 내에 있는 GetNotifyName 코드
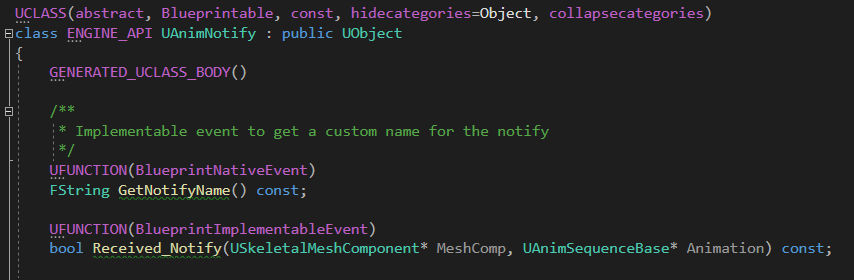
AnimNotify.cpp 내에 있는 GetNotifyName_Implementation 코드
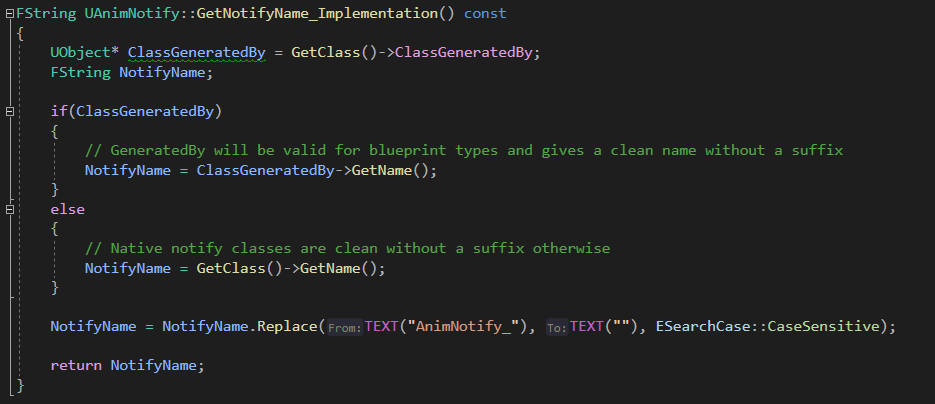
ICharacter 생성
새 c++ 클래스 - Unreal Interface - ICharacter 생성
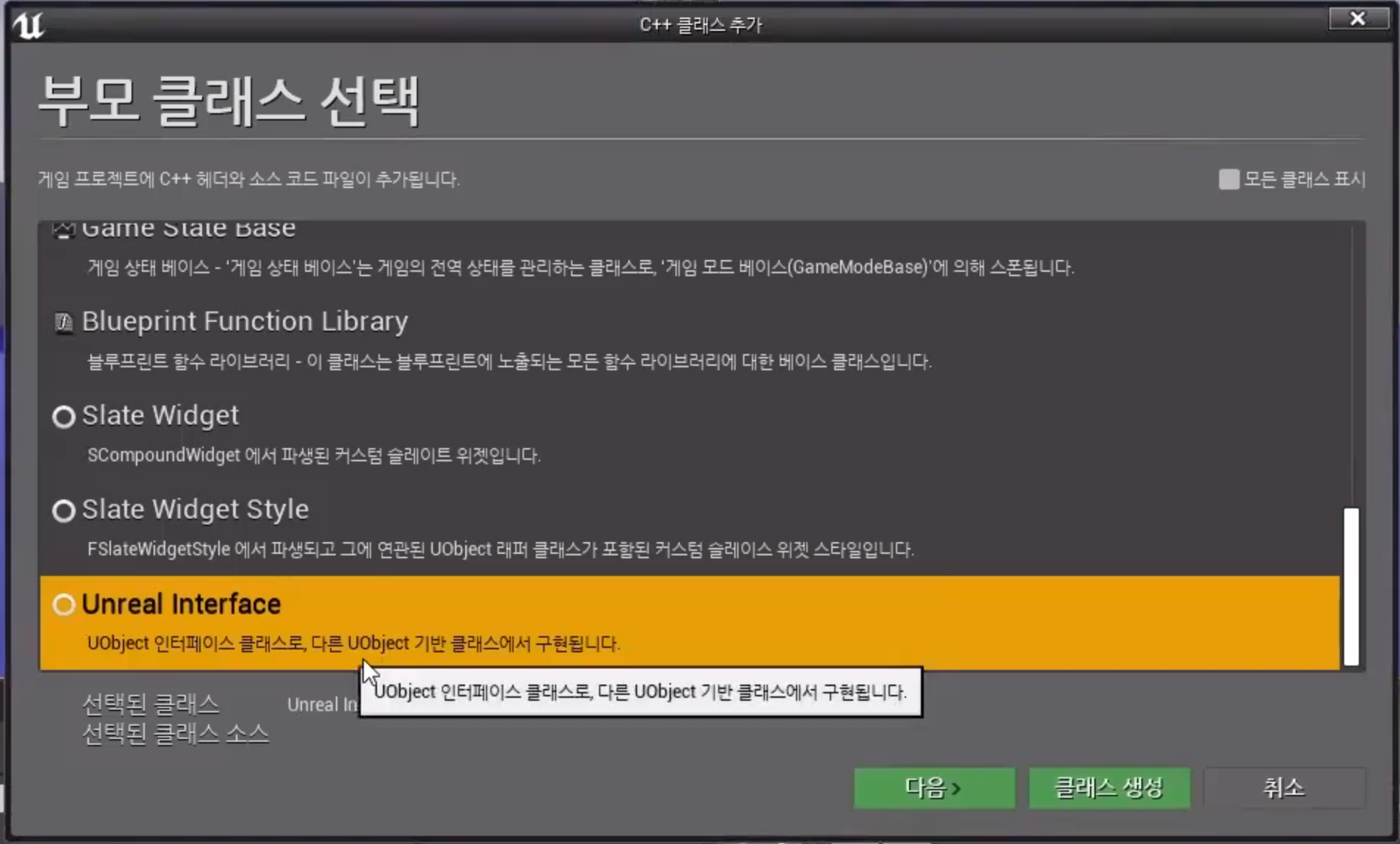
ICharacter.h
#pragma once #include "CoreMinimal.h" #include "UObject/Interface.h" #include "ICharacter.generated.h" UINTERFACE(MinimalAPI) //직렬화를 위한 부분 class UICharacter : public UInterface { GENERATED_BODY() }; class U2212_06_API IICharacter { GENERATED_BODY() public: virtual void End_BackStep() { } };
ICharacter.cpp
#include "Characters/ICharacter.h"
Backstep_Montage에 AnimNotify_EndState 할당
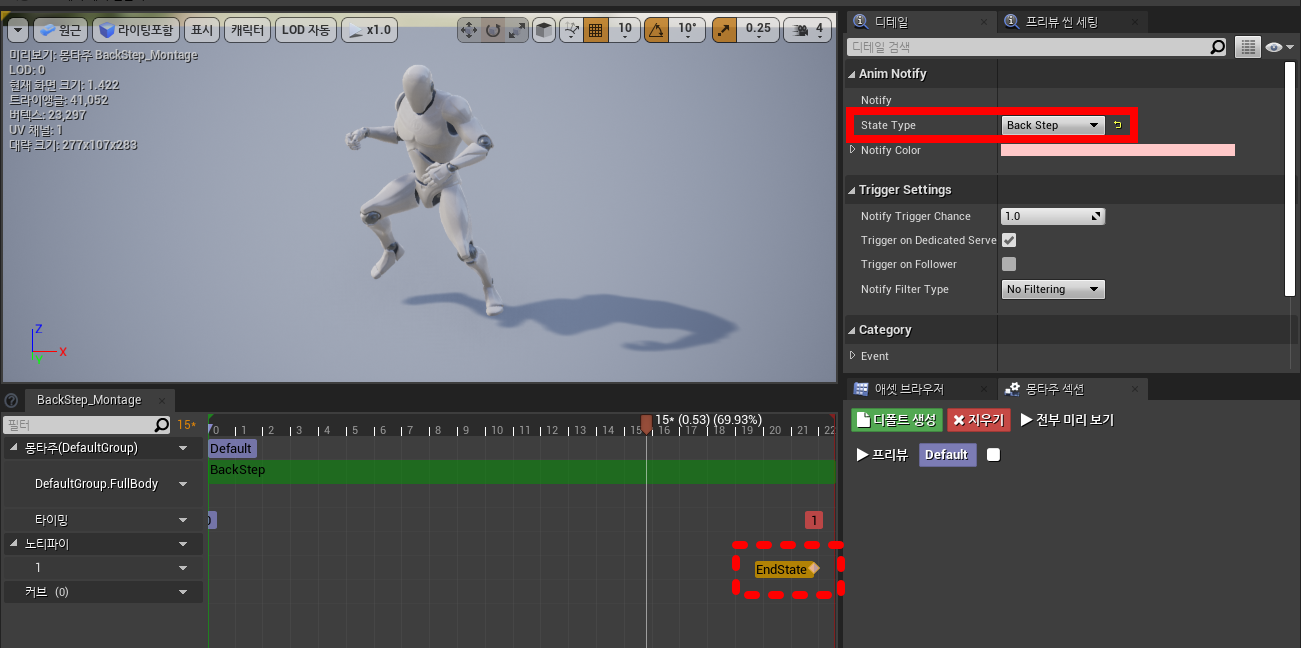
실행화면
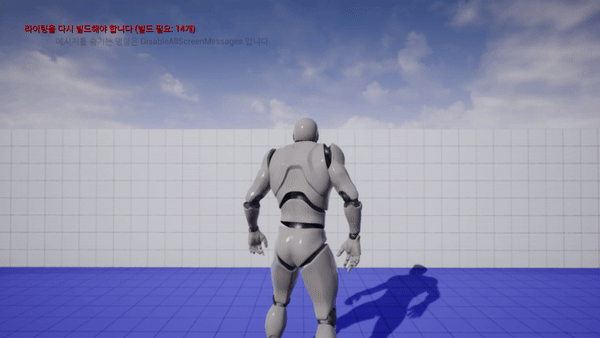
Weapon System 시작
Weapon System 구조 개요
UDataAsset
↑
UCWeaponAssets
WeaponComponent
ACAttachment
- 모양을 결정해줄 클래스
UCEquipment
- 장착방식.
- ex. 어떤 몽타주에서 장착할지. 몽타주 없이 장착할지
UCDoAction
위의 구조를 지켜 무기 시스템을 만들것이다. Slate UI를 함께 사용하여 구현할 것이다.
CAttachment 생성
새 c++ 클래스 - Actor - CAttachment생성
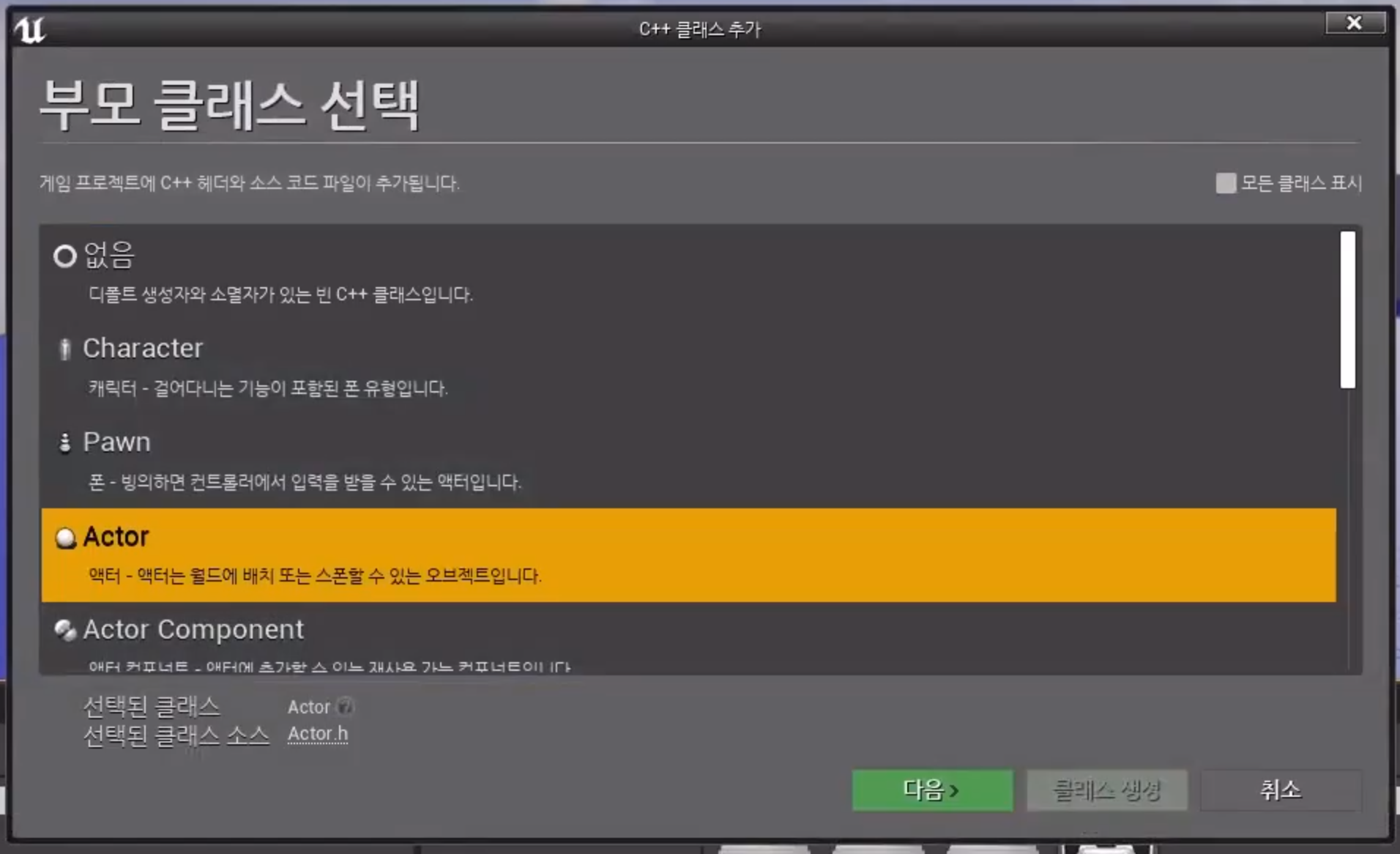
CAttachment.h
#pragma once #include "CoreMinimal.h" #include "GameFramework/Actor.h" #include "CAttachment.generated.h" UCLASS() class U2212_06_API ACAttachment : public AActor { GENERATED_BODY() protected: UPROPERTY(BlueprintReadOnly, VisibleAnywhere) class USceneComponent* Root; public: ACAttachment(); protected: virtual void BeginPlay() override; protected: UPROPERTY(BlueprintReadOnly, Category = "Game") class ACharacter* OwnerCharacter; };
CAttachment.cpp
#include "Weapons/CAttachment.h" #include "Global.h" #include "GameFramework/Character.h" #include "Components/SceneComponent.h" ACAttachment::ACAttachment() { CHelpers::CreateComponent(this, &Root, "Root"); } void ACAttachment::BeginPlay() { OwnerCharacter = Cast<ACharacter>(GetOwner()); //ACharacter를 먼저 Cast 한 후에 Super::BeginPlay() 호출. Super::BeginPlay(); }
BP_CAttachment_Sword 생성

CWeaponAsset 생성
새 c++ 클래스 - Data Asset - CWeaponAsset 생성
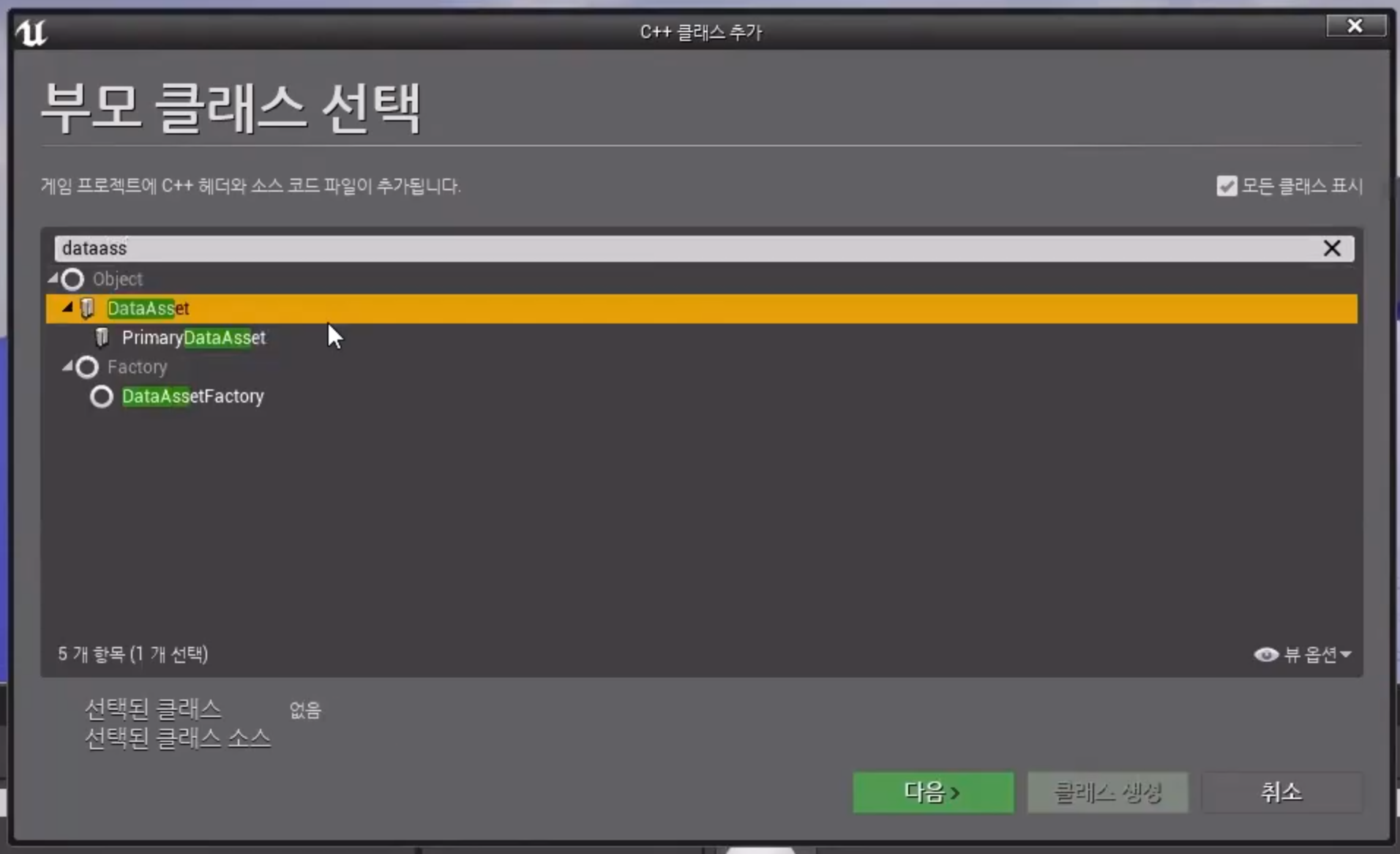
CWeaponAsset.h
#pragma once #include "CoreMinimal.h" #include "Engine/DataAsset.h" #include "CWeaponAsset.generated.h" UCLASS() class U2212_06_API UCWeaponAsset : public UDataAsset { GENERATED_BODY() private: UPROPERTY(EditAnywhere) TSubclassOf<class ACAttachment> AttachmentClass; public: FORCEINLINE class ACAttachment* GetAttachment() { return Attachment; }//외부에 생성된 것을 리턴해줌. public: UCWeaponAsset(); void BeginPlay(class ACharacter* InOwner); private: //UPROPERTY를 붙여 가비지 콜렉터가 제거하기 전까지 물고 있게 만든다. //UWeaponAsset은 UObject로부터 상속받아 Actor?의 생성주기에 영향을 받지 않아 가비지 콜렉터에 영향을 받는다. UPROPERTY() class ACAttachment* Attachment; };
UPROPERTY를 붙여 가비지 콜렉터가 제거하기 전까지 물고 있게 만든다.
UWeaponAsset은 UObject로부터 상속받아 Actor?의 생성주기에 영향을 받지 않아 가비지 콜렉터에 영향을 받는다.
CWeaponAsset.cpp
#include "Weapons/CWeaponAsset.h" #include "Global.h" #include "CAttachment.h" #include "GameFramework/Character.h" UCWeaponAsset::UCWeaponAsset() { AttachmentClass = ACAttachment::StaticClass(); } void UCWeaponAsset::BeginPlay(ACharacter* InOwner) { if (!!AttachmentClass)//AttachmentClass가 선택이 되어 있다면 { FActorSpawnParameters params; params.Owner = InOwner; Attachment = InOwner->GetWorld()->SpawnActor<ACAttachment>(AttachmentClass, params); } }
DA_Sword 생성
기타 - 데이터 에셋 - CWeaponAsset - DA_Sword 생성
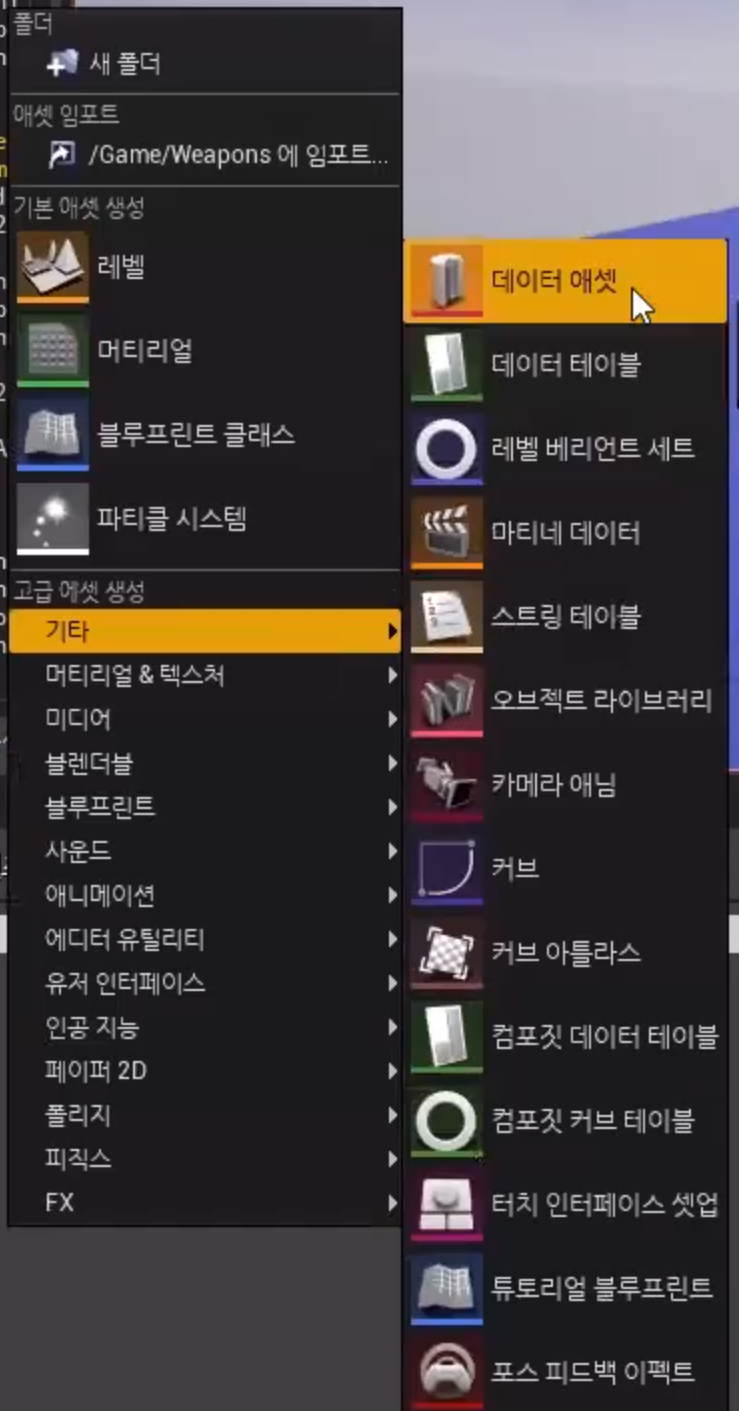
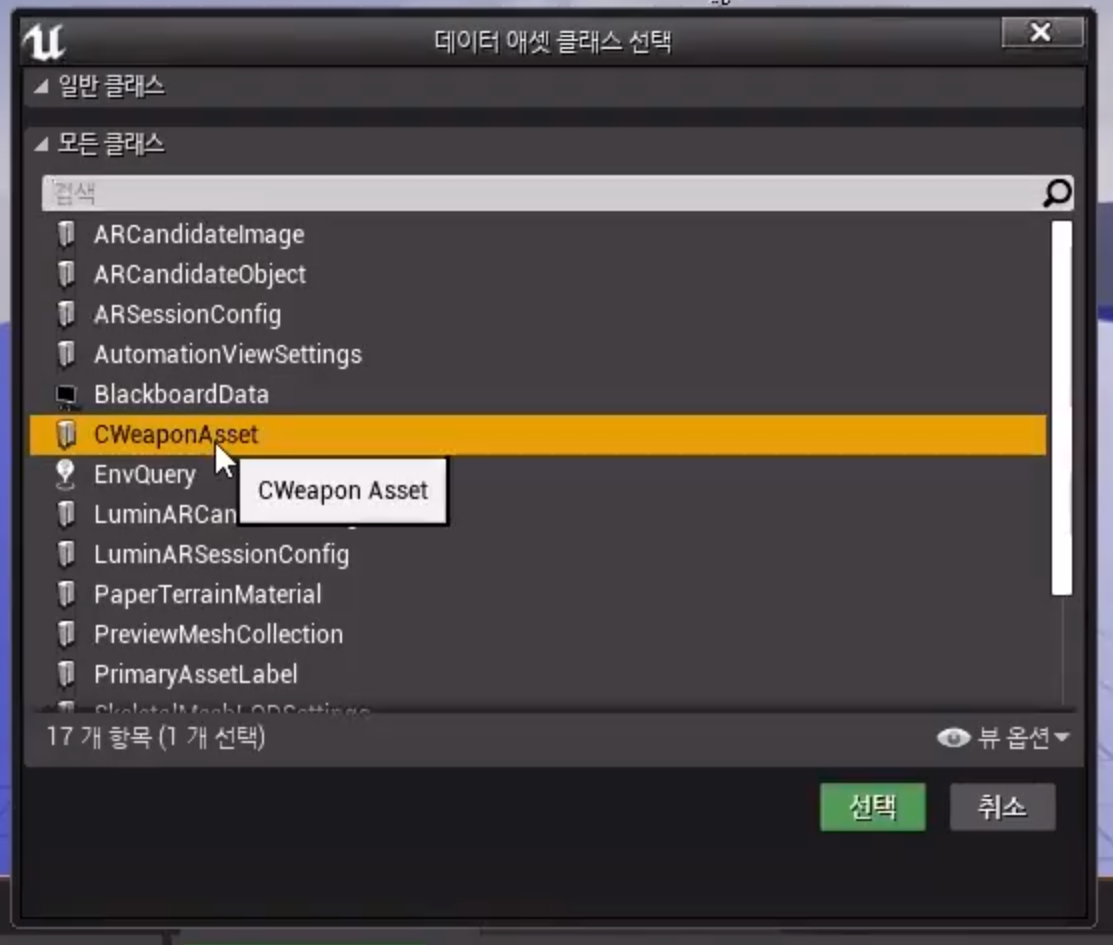
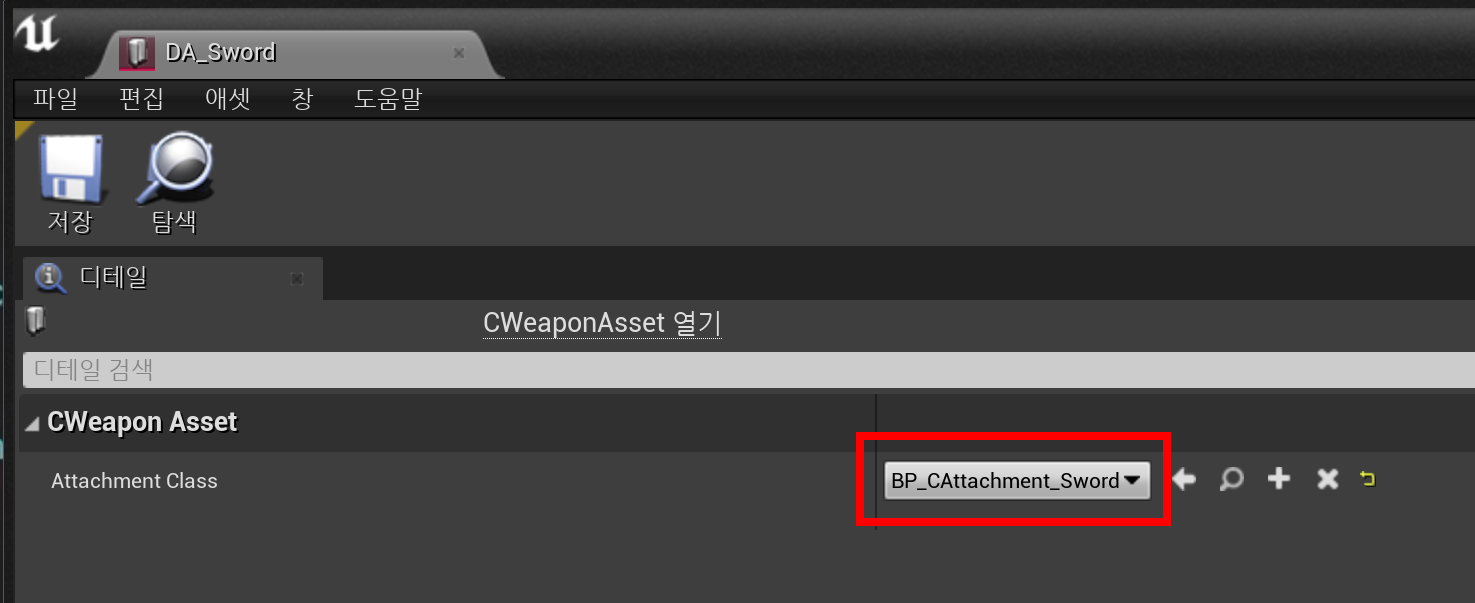
Attachment Class에 BP_CAttachment_Sword 할당
'⭐ Unreal Engine > UE RPG Weapon System' 카테고리의 다른 글
[UE] AnimNotify, DoAction (0) | 2023.05.08 |
---|---|
[UE] 무기 장착 및 기본 공격하기 (0) | 2023.05.02 |
[UE] 무기 시스템 설계하기 (0) | 2023.05.01 |
[UE] Component 컴포넌트, Player 이동 (0) | 2023.04.27 |
[UE] Player 초기 세팅하기 (0) | 2023.04.26 |
댓글
이 글 공유하기
다른 글
-
[UE] 무기 장착 및 기본 공격하기
[UE] 무기 장착 및 기본 공격하기
2023.05.02 -
[UE] 무기 시스템 설계하기
[UE] 무기 시스템 설계하기
2023.05.01 -
[UE] Component 컴포넌트, Player 이동
[UE] Component 컴포넌트, Player 이동
2023.04.27 -
[UE] Player 초기 세팅하기
[UE] Player 초기 세팅하기
2023.04.26
댓글을 사용할 수 없습니다.